Welcome
Remade-AI/ Cartoon_Jaw_Drop
API Sample: Remade-AI/Cartoon_Jaw_Drop
You don't have any projects yet. To be able to use our api service effectively, please sign in/up and create a project.
Get your api keyPrepare Authentication Signature
//Sign up Wiro dashboard and create project
export YOUR_API_KEY="{{useSelectedProjectAPIKey}}";
export YOUR_API_SECRET="XXXXXXXXX";
//unix time or any random integer value
export NONCE=$(date +%s);
//hmac-SHA256 (YOUR_API_SECRET+Nonce) with YOUR_API_KEY
export SIGNATURE="$(echo -n "${YOUR_API_SECRET}${NONCE}" | openssl dgst -sha256 -hmac "${YOUR_API_KEY}")";
Create a New Folder - Make HTTP Post Request
Create a New Folder - Response
Upload a File to the Folder - Make HTTP Post Request
Upload a File to the Folder - Response
Run Command - Make HTTP Post Request
curl -X POST "{{apiUrl}}/Run/{{toolSlugOwner}}/{{toolSlugProject}}" \
-H "Content-Type: {{contentType}}" \
-H "x-api-key: ${YOUR_API_KEY}" \
-H "x-nonce: ${NONCE}" \
-H "x-signature: ${SIGNATURE}" \
-d '{{toolParameters}}';
Run Command - Response
//response body
{
"errors": [],
"taskid": "2221",
"socketaccesstoken": "eDcCm5yyUfIvMFspTwww49OUfgXkQt",
"result": true
}
Get Task Detail - Make HTTP Post Request
curl -X POST "{{apiUrl}}/Task/Detail" \
-H "Content-Type: {{contentType}}" \
-H "x-api-key: ${YOUR_API_KEY}" \
-H "x-nonce: ${NONCE}" \
-H "x-signature: ${SIGNATURE}" \
-d '{
"tasktoken": 'eDcCm5yyUfIvMFspTwww49OUfgXkQt',
}';
Get Task Detail - Response
//response body
{
"total": "1",
"errors": [],
"tasklist": [
{
"id": "2221",
"uuid": "15bce51f-442f-4f44-a71d-13c6374a62bd",
"name": "",
"socketaccesstoken": "eDcCm5yyUfIvMFspTwww49OUfgXkQt",
"parameters": {
"inputImage": "https://api.wiro.ai/v1/File/mCmUXgZLG1FNjjjwmbtPFr2LVJA112/inputImage-6060136.png"
},
"debugoutput": "",
"debugerror": "",
"starttime": "1734513809",
"endtime": "1734513813",
"elapsedseconds": "6.0000",
"status": "task_postprocess_end",
"cps": "0.000585000000",
"totalcost": "0.003510000000",
"guestid": null,
"projectid": "699",
"modelid": "598",
"description": "",
"basemodelid": "0",
"runtype": "model",
"modelfolderid": "",
"modelfileid": "",
"callbackurl": "",
"marketplaceid": null,
"createtime": "1734513807",
"canceltime": "0",
"assigntime": "1734513807",
"accepttime": "1734513807",
"preprocessstarttime": "1734513807",
"preprocessendtime": "1734513807",
"postprocessstarttime": "1734513813",
"postprocessendtime": "1734513814",
"pexit": "0",
"categories": "["tool","image-to-image","quick-showcase","compare-landscape"]",
"outputs": [
{
"id": "6bc392c93856dfce3a7d1b4261e15af3",
"name": "0.png",
"contenttype": "image/png",
"parentid": "6c1833f39da71e6175bf292b18779baf",
"uuid": "15bce51f-442f-4f44-a71d-13c6374a62bd",
"size": "202472",
"addedtime": "1734513812",
"modifiedtime": "1734513812",
"accesskey": "dFKlMApaSgMeHKsJyaDeKrefcHahUK",
"foldercount": "0",
"filecount": "0",
"ispublic": 0,
"expiretime": null,
"url": "https://cdn1.wiro.ai/6a6af820-c5050aee-40bd7b83-a2e186c6-7f61f7da-3894e49c-fc0eeb66-9b500fe2/0.png"
}
],
"size": "202472"
}
],
"result": true
}
Get Task Process Information and Results with Socket Connection
<script type="text/javascript">
window.addEventListener('load',function() {
//Get socketAccessToken from task run response
var SocketAccessToken = 'eDcCm5yyUfIvMFspTwww49OUfgXkQt';
WebSocketConnect(SocketAccessToken);
});
//Connect socket with connection id and register task socket token
async function WebSocketConnect(accessTokenFromAPI) {
if ("WebSocket" in window) {
var ws = new WebSocket("wss://socket.wiro.ai/v1");
ws.onopen = function() {
//Register task socket token which has been obtained from task run API response
ws.send('{"type": "task_info", "tasktoken": "' + accessTokenFromAPI + '"}');
};
ws.onmessage = function (evt) {
var msg = evt.data;
try {
var debugHtml = document.getElementById('debug');
debugHtml.innerHTML = debugHtml.innerHTML + "\n" + msg;
var msgJSON = JSON.parse(msg);
console.log('msgJSON: ', msgJSON);
if(msgJSON.type != undefined)
{
console.log('msgJSON.target: ',msgJSON.target);
switch(msgJSON.type) {
case 'task_queue':
console.log('Your task has been waiting in the queue.');
break;
case 'task_accept':
console.log('Your task has been accepted by the worker.');
break;
case 'task_preprocess_start':
console.log('Your task preprocess has been started.');
break;
case 'task_preprocess_end':
console.log('Your task preprocess has been ended.');
break;
case 'task_assign':
console.log('Your task has been assigned GPU and waiting in the queue.');
break;
case 'task_start':
console.log('Your task has been started.');
break;
case 'task_output':
console.log('Your task has been started and printing output log.');
console.log('Log: ', msgJSON.message);
break;
case 'task_error':
console.log('Your task has been started and printing error log.');
console.log('Log: ', msgJSON.message);
break;
case 'task_output_full':
console.log('Your task has been completed and printing full output log.');
break;
case 'task_error_full':
console.log('Your task has been completed and printing full error log.');
break;
case 'task_end':
console.log('Your task has been completed.');
break;
case 'task_postprocess_start':
console.log('Your task postprocess has been started.');
break;
case 'task_postprocess_end':
console.log('Your task postprocess has been completed.');
console.log('Outputs: ', msgJSON.message);
//output files will add ui
msgJSON.message.forEach(function(currentValue, index, arr){
console.log(currentValue);
var filesHtml = document.getElementById('files');
filesHtml.innerHTML = filesHtml.innerHTML + '<img src="' + currentValue.url + '" style="height:300px;">'
});
break;
}
}
} catch (e) {
console.log('e: ', e);
console.log('msg: ', msg);
}
};
ws.onclose = function() {
alert("Connection is closed...");
};
} else {
alert("WebSocket NOT supported by your Browser!");
}
}
</script>
Prepare UI Elements Inside Body Tag
<div id="files"></div>
<pre id="debug"></pre>
Cartoon Jaw Drop LoRA for Wan2.1 14B I2V 480p
Overview
This LoRA is trained on the Wan2.1 14B I2V 480p model and allows you to apply the Cartoon Jaw Drop effect to images!
Features
- Trained on the Wan2.1 14B 480p I2V base model
- Consistent results across different object types
- Simple prompt structure that's easy to adapt
Community
- Discord: Join our community to generate videos with this LoRA for free
- Request LoRAs: We're training and open-sourcing Wan2.1 LoRAs for free - join our Discord to make requests!
- Prompt
- The video shows Pluto the dog, wearing a red collar, who is smiling wide, then his mouth transforms into a dr0p_j88 comical jaw drop, extending down in a long, rectangular shape, and revealing his tongue and teeth.
- Prompt
- The video shows a man with a neutral expression. Suddenly his jaw extremely widens to create a dr0p_j88 comical jaw drop.
- Prompt
- The video shows the man with a subtle smile, then suddenly his mouth drops open wide in a dr0p_j88 comical jaw drop, showing his teeth and tongue.
Model File and Inference Workflow
📥 Download Links:
- cartoon_jaw_drop_50_epochs.safetensors - LoRA Model File
- wan_img2vid_lora_workflow.json - Wan I2V with LoRA Workflow for ComfyUI
Recommended Settings
- LoRA Strength: 1.0
- Embedded Guidance Scale: 6.0
- Flow Shift: 5.0
Trigger Words
The key trigger phrase is: dr0p_j88 comical jaw drop
Prompt Template
For prompting, check out the example prompts; this way of prompting seems to work very well.
ComfyUI Workflow
This LoRA works with a modified version of Kijai's Wan Video Wrapper workflow. The main modification is adding a Wan LoRA node connected to the base model.

See the Downloads section above for the modified workflow.
Model Information
The model weights are available in Safetensors format. See the Downloads section above.
Training Details
- Base Model: Wan2.1 14B I2V 480p
- Training Data: Trained on 50 seconds of video comprised of 12 short clips (each clip captioned separately) of cartoon jaw drops.
- Epochs: 5
Acknowledgments
Special thanks to Kijai for the ComfyUI Wan Video Wrapper and tdrussell for the training scripts!
Tools
View All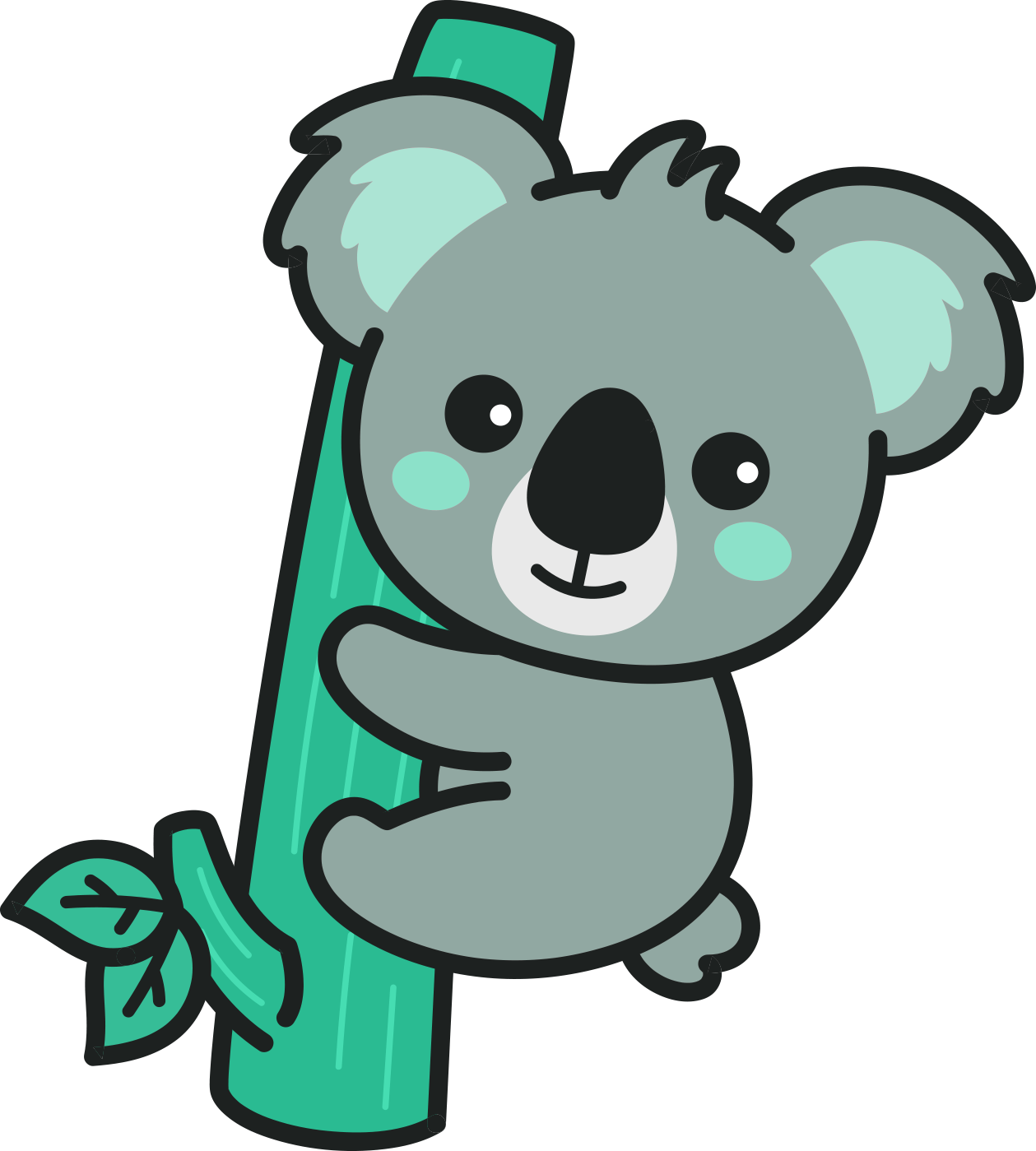
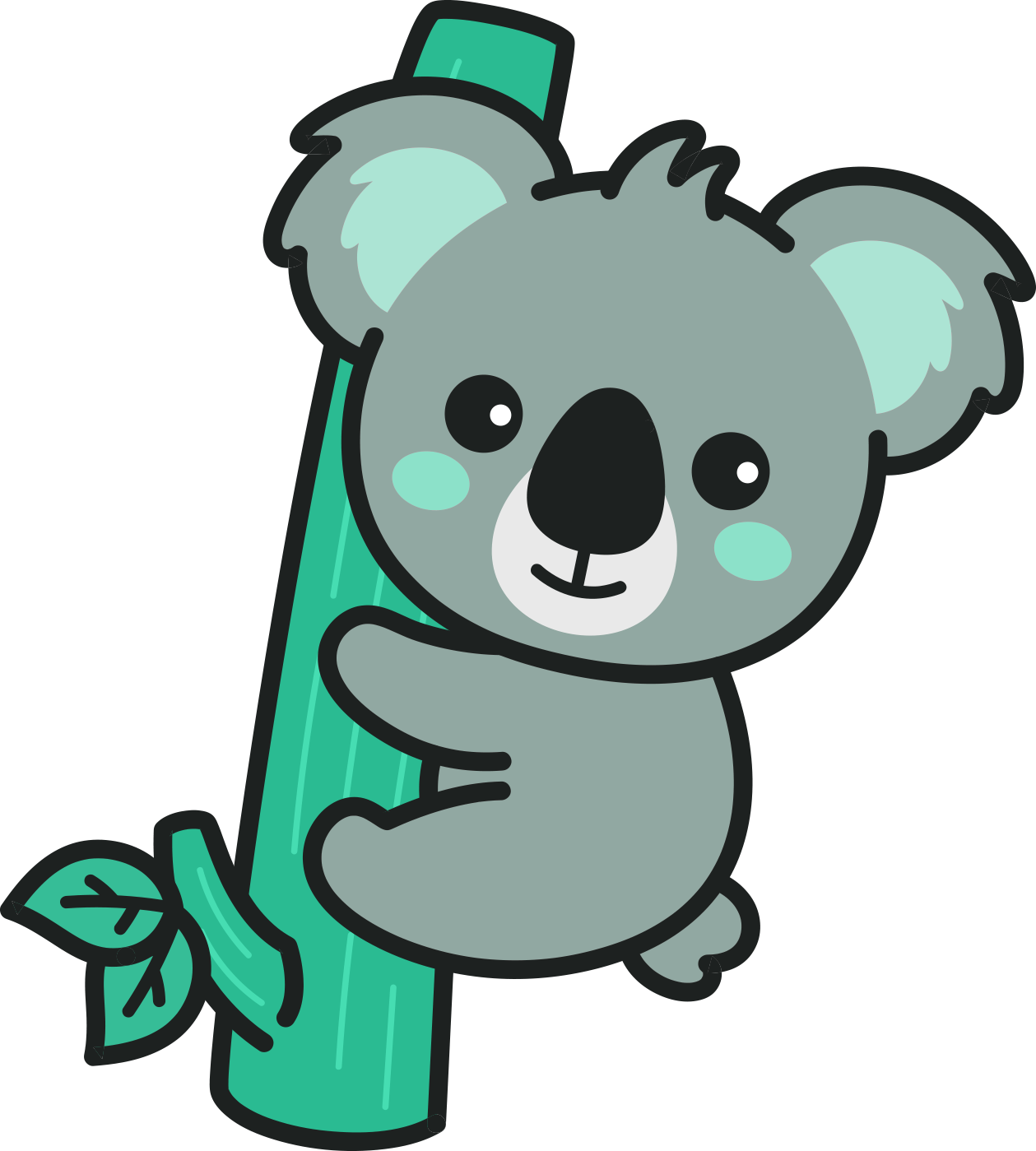